Java does it pretty well. C++ sucks by default. What is that?: The answer: Backtraces.
Sometimes assert information (file name and line number where assertion occured is not enough to guess where the problem is. It's useful to see the backtrace (stack trace in Java vocabulary) of calling methods/functions. You can achieve that in C++ using gdb (and analysing generated core files or run the program under debugger), but it's not a "light" (and elegant) solution (especially for embedded systems).
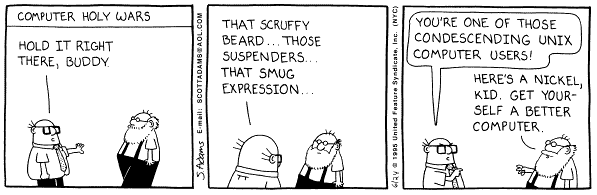
I'm presenting here a method to collect as meaningful backtraces in C++ as possible. Just link to module below and you will see pretty backtraces on abort/assert/uncaught throw/...
Let's first, install some handlers to connect to interesting events in program:
static int install_handler() {
struct sigaction sigact;
sigact.sa_flags = SA_SIGINFO | SA_ONSTACK;
sigact.sa_sigaction = segv_handler;
if (sigaction(SIGSEGV, &sigact, (struct sigaction *)NULL) != 0) {
fprintf(stderr, "error setting signal handler for %d (%s)\n",
SIGSEGV, strsignal(SIGSEGV));
}
sigact.sa_sigaction = abrt_handler;
if (sigaction(SIGABRT, &sigact, (struct sigaction *)NULL) != 0) {
fprintf(stderr, "error setting signal handler for %d (%s)\n",
SIGABRT, strsignal(SIGABRT));
}
std::set_terminate(terminate_handler);
std::set_unexpected(unexpected_handler);
qInstallMsgHandler(qt_message_handler);
return 0;
}
static int x = install_handler();
Some notes:
- qInstallHandler: some QT-related exceptions were not properly reported as backtrace, used for Q_ASSERT
- set_terminate, set_unexpected: C++ standard of "catching" uncaught exceptions
- sigaction: catch assert() macro call
Then define the handlers itself:
static void segv_handler(int, siginfo_t*, void*) {
print_stacktrace("segv_handler");
exit(1);
}
static void abrt_handler(int, siginfo_t*, void*) {
print_stacktrace("abrt_handler");
exit(1);
}
static void terminate_handler() {
print_stacktrace("terminate_handler");
}
static void unexpected_handler() {
print_stacktrace("unexpected_handler");
}
static void qt_message_handler(QtMsgType type, const char *msg) {
fprintf(stderr, "%s\n", msg);
switch (type) {
case QtDebugMsg:
case QtWarningMsg:
break;
case QtCriticalMsg:
case QtFatalMsg:
print_stacktrace("qt_message_handler");
}
}
And finally define function that renders stacktrace to file handle passed:
/** Print a demangled stack backtrace of the caller function to FILE* out. */
static void print_stacktrace(const char* source, FILE *out = stderr, unsigned int max_frames = 63)
{
char linkname[512]; /* /proc/
/exe */
char buf[512];
pid_t pid;
int ret;
/* Get our PID and build the name of the link in /proc */
pid = getpid();
snprintf(linkname, sizeof(linkname), "/proc/%i/exe", pid);
/* Now read the symbolic link */
ret = readlink(linkname, buf, 512);
buf[ret] = 0;
fprintf(out, "stack trace (%s) for process %s (PID:%d):\n",
source, buf, pid);
// storage array for stack trace address data
void* addrlist[max_frames+1];
// retrieve current stack addresses
int addrlen = backtrace(addrlist, sizeof(addrlist) / sizeof(void*));
if (addrlen == 0) {
fprintf(out, " \n");
return;
}
// resolve addresses into strings containing "filename(function+address)",
// this array must be free()-ed
char** symbollist = backtrace_symbols(addrlist, addrlen);
// allocate string which will be filled with the demangled function name
size_t funcnamesize = 256;
char* funcname = (char*)malloc(funcnamesize);
// iterate over the returned symbol lines. skip first two,
// (addresses of this function and handler)
for (int i = 2; i < addrlen; i++)
{
char *begin_name = 0, *begin_offset = 0, *end_offset = 0;
// find parentheses and +address offset surrounding the mangled name:
// ./module(function+0x15c) [0x8048a6d]
for (char *p = symbollist[i]; *p; ++p)
{
if (*p == '(')
begin_name = p;
else if (*p == '+')
begin_offset = p;
else if (*p == ')' && begin_offset) {
end_offset = p;
break;
}
}
if (begin_name && begin_offset && end_offset
&& begin_name < begin_offset)
{
*begin_name++ = '\0';
*begin_offset++ = '\0';
*end_offset = '\0';
// mangled name is now in [begin_name, begin_offset) and caller
// offset in [begin_offset, end_offset). now apply
// __cxa_demangle():
int status;
char* ret = abi::__cxa_demangle(begin_name,
funcname, &funcnamesize, &status);
if (status == 0) {
funcname = ret; // use possibly realloc()-ed string
fprintf(out, " (PID:%d) %s : %s+%s\n",
pid, symbollist[i], funcname, begin_offset);
}
else {
// demangling failed. Output function name as a C function with
// no arguments.
fprintf(out, " (PID:%d) %s : %s()+%s\n",
pid, symbollist[i], begin_name, begin_offset);
}
}
else
{
// couldn't parse the line? print the whole line.
fprintf(out, " (PID:%d) %s: ??\n", pid, symbollist[i]);
}
}
free(funcname);
free(symbollist);
fprintf(out, "stack trace END (PID:%d)\n", pid);
}
And finally: some includes to be able to compile the module:
#include <cxxabi.h>
#include <execinfo.h>
#include <execinfo.h>
#include <signal.h>
#include <stdio.h>
#include <stdio.h>
#include <stdlib.h>
#include <stdlib.h>
#include <string.h>
#include <ucontext.h>
#include <unistd.h>
#include <Qt/qapplication.h>
Note that you have to pass some flags during compiling / linking phase in order to have dynamic backtraces available:
-funwind-tables for compiler
- -rdynamic for linker